You know that moment when you stare at a blinking cursor and wonder where to even start? Coding can feel like that—overwhelming and confusing. But when you break it down into real steps, it’s way more doable. This isn’t about memorizing some boring rules. It’s about having a game plan so you’re not lost every time you start a coding project.
Most people think coding is just typing out commands, but that’s only a tiny part. Everything starts with a crystal clear idea of what you actually need to build. If you rush into writing code before you really get what the program should do—good luck making it work later. Even professional programmers get tripped up if they skip this part.
Here’s the real deal: coding is a series of steps that help you turn ideas into something that works. Miss one of these steps, and you’re probably spending twice the time fixing things. Stick with these steps, and you’ll make way fewer mistakes (plus, your future self will thank you later).
- Figuring Out What You Need to Build
- Breaking It Down: Planning Your Code
- Choosing the Right Tools and Language
- Writing the Code
- Debugging: Fixing Mistakes
- Testing Your Program
- Keeping Your Code Fresh and Updated
Figuring Out What You Need to Build
This is where everything kicks off. Before you even think about writing a single line of code, you have to know what you’re building. It sounds obvious, but it’s the step everyone rushes through and later regrets. Take your time with this.
First, talk to whoever needs the program — or, if it’s just for you, clearly write out what you want it to do. Don’t worry about how to build it yet. Just nail down exactly what problem you’re trying to solve. Grab a notebook, open a note app, or draw it on a napkin—just don’t skip this part.
- List out all the things your program should do. Don’t get fancy with the tech talk. Use plain language.
- Sketch what the screen should look like if there is a user interface. Boxes, buttons, whatever you need—be messy, just get the idea down.
- Write out a couple of possible scenarios. For example, if you’re building a to-do list app, write how someone adds a task, marks it done, or deletes it.
If you’re working with someone else, ask a ton of questions until you’re both on the same page. Developers at big companies spend days (sometimes weeks) chopping up these requirements before starting any coding. Otherwise, you’ll end up with a program that doesn’t do what anyone wanted in the first place.
This early step makes all the later stages way easier. You won’t waste hours coding something only to find out it’s not what was needed. If you ever feel lost during the process, come back and check your notes. You’d be surprised how much going back to this coding steps checklist saves time and headaches later.
Breaking It Down: Planning Your Code
Before you start typing anything, you need a plan. It sounds basic, but skipping this step is where beginners hit most of their roadblocks. You can't just jump in with a vague idea and hope the code will magically work out.
Start by dividing your big task into smaller, manageable parts. Programmers call this "decomposition." Imagine building a mobile app: instead of thinking about the whole app, break it down into screens, buttons, and features. Each small part becomes a mini-task to hit one by one. This saves you from feeling overwhelmed and makes it easier to spot what you’ll need to research or ask about.
A good trick is to write out your plan on paper or use tools like flowcharts and pseudocode (writing the logic in plain English). This helps you figure out the order of steps and spot anything confusing before you start coding. Some beginners use sticky notes for each step—sounds simple, but it works.
Here’s a simple way to plan any coding steps:
- Write down what your program needs to do (the requirements).
- Break the main task into smaller steps or modules.
- Decide what data you’ll need and how you’ll get and use it.
- Draw out the main logic or flow—either as a flowchart or with bullet points.
- Spot problems or tricky parts before you start coding—Google if you need help.
Did you know that teams using detailed plans cut their coding time in half? Stack Overflow ran a survey in 2022 showing that coders who sketch out steps make 45% fewer errors in the first version of their programs.
Planning Tool | Why It Helps |
---|---|
Flowchart | Visualizes steps so you don’t miss any. |
Pseudocode | Lets you check logic in plain words. |
Sticky Notes | Easy to arrange and rearrange steps. |
If your plan feels confusing, break it down even further. Always start coding with at least some kind of map—otherwise, you’re just guessing and hoping for the best.
Choosing the Right Tools and Language
This is where things can get confusing, because there are a million choices—Python, JavaScript, C++, and so many more. Picking the right one depends on what you want to build. For simple apps or automating tasks, Python is almost always the easiest place to start. If you want to build websites, JavaScript is the main player. Making mobile apps? People often go with Swift (for iOS) or Kotlin (for Android).
Here’s a quick cheat sheet to help you decide:
- Python: Super beginner-friendly. Great for data analysis, automation, and quick prototypes.
- JavaScript: The go-to for websites and anything that runs in a browser.
- Java: Big for Android apps and enterprise software.
- C++: Used in games, high-performance apps, and situations where speed really matters.
- Swift/Kotlin: The must-haves for iOS and Android mobile development.
But the language is just half the story. The right tools can save you hours of hassle. An IDE (Integrated Development Environment) like VS Code is super popular because it works with almost any language and has smart features that catch errors while you type. For web stuff, Chrome’s DevTools can show you exactly why your site is misbehaving. If you work with Python, Jupyter Notebooks make experiment-ing with code much less scary.
Don’t waste time trying to learn every tool at once. Pick the basic tools for your project, learn them well, and expand from there. And remember—many pro coders still end up Googling how to do things. That’s normal, not a sign you’re doing something wrong. Stick with your chosen tools and language, and you’ll ramp up faster and build better stuff. If you're looking for the right coding classes, make sure they teach you real-world tools, not just theory. That’s where the coding steps really start to feel practical.
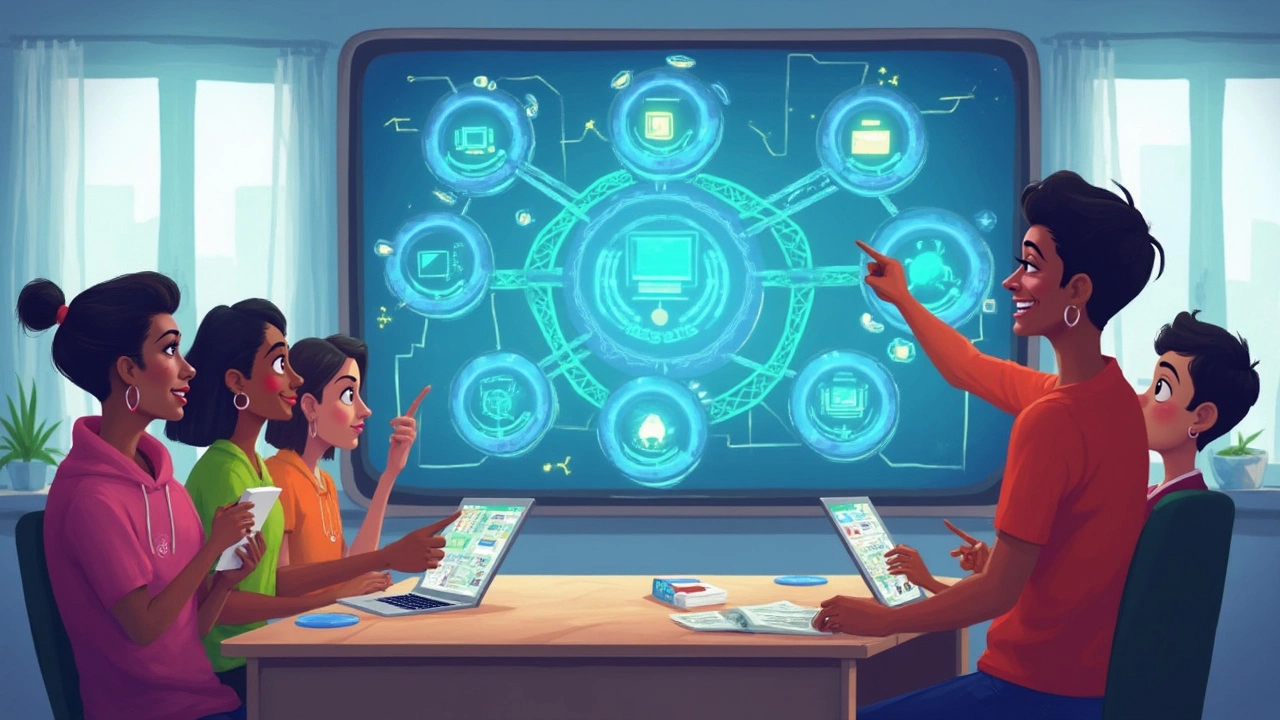
Writing the Code
This is the part where everyone pictures programmers tapping away at a keyboard. But it's not just about typing fast. It’s about translating the plan you made into actual instructions the computer understands. If you skip prep and jump straight in, you’ll likely end up backtracking a lot.
Start by opening your chosen code editor—Visual Studio Code is super popular for beginners and pros, but others like PyCharm or even repl.it online work well too. Make sure you’ve set up your workspace with the right language and tools for your project.
One trick: write your code in small chunks. Don’t try to do everything at once. Build a piece, run it, and make sure it works before moving on. If you get stuck, there’s no shame in Googling error messages. Actually, Stack Overflow ran a survey in 2023 showing over 85% of professional developers search for coding fixes online at least once a day.
- Write clear, simple code first. Fancy isn’t better, working is better.
- Comment your code, even if you’re the only one reading it. (Future you will forget why you did something, trust me.)
- Use meaningful names for variables and functions.
score
beatsx1
every time. - Test as you go, not just when you finish the whole thing.
Here’s a quick look at popular languages and what new coders usually pick them for:
Language | Common Uses | Why Beginners Like It |
---|---|---|
Python | Web, data, basics | Simple syntax, easy to read |
JavaScript | Web pages, apps | Immediate browser feedback |
Java | Android, bigger apps | Structured and widely used |
Scratch | Basic learning, kids | Visual and drag-and-drop |
Don’t be afraid to experiment. Every good coder has a folder of messy early code. You get better by actually doing it, so aim for progress, not perfection. If you stick to the coding steps, your projects will not only work—they’ll make sense when you come back to them next month.
Debugging: Fixing Mistakes
No one writes perfect code on the first try. Bugs happen—even the pros deal with them daily. Debugging is all about finding and fixing those pesky errors that stop your program from working right. This step saves you from hours of confusion down the road.
Most of the time, mistakes fall into a few categories: typos, logic errors, or using things the wrong way. The first thing to do is read the error messages. Don’t just ignore them—they usually point you in the right direction. A recent 2024 survey by Stack Overflow found that around 42% of coding time is spent just on debugging. So if you’re stuck, you’re not alone.
- Start by isolating the problem. Make small changes and test as you go. This way, you know exactly when something broke.
- Print things out in your code (“print statements” are your best friends) to see what’s actually happening.
- Use the built-in debugger in your editor. Even basic text editors have simple options to step through your code line by line.
- Double-check for common issues: missing brackets, spelling errors, or accidentally using the wrong variable.
- If you get stuck, explain the issue out loud or to someone else (“rubber duck debugging”). Saying it makes you notice things you missed.
If you’re curious about how much debugging impacts productivity, check out this breakdown:
Activity | Percent of Coding Time |
---|---|
Writing New Code | 38% |
Debugging | 42% |
Testing Code | 14% |
Other Tasks | 6% |
Some bugs can take minutes to fix; others eat up days. Don’t get frustrated—every mistake you find is one less thing to worry about later. And the more you debug, the better you get at catching mistakes early.
Testing Your Program
This is the part where you find out if your code actually does what you wanted—or if it's just going to give you random errors. Testing your program might sound basic, but skipping it is a recipe for headaches. Making sure your program works gets even more important if anyone else is going to use it, or if you want to keep bugs from piling up later.
There are a few types of testing you’ll see pop up all the time:
- Manual testing: This is just you, running your program and clicking around or trying different inputs to see what happens. If you’re building a calculator app, you might try entering all kinds of funny numbers to see if it breaks.
- Automated testing: Here, you actually write code whose only job is to test your main code. Things like unit tests let you check if each small part (like a single function) does what it’s supposed to. Frameworks like Jest (for JavaScript) or PyTest (for Python) make this easier—and once you set them up, they catch a lot of silly mistakes before you ever hit 'launch.'
- User testing: Have someone who wasn’t involved in writing the code try it out. If they run into problems or get confused, you probably missed something.
Don’t know where to start? Try this checklist:
- Write simple cases: Test your program with normal, expected inputs.
- Try weird stuff: Enter wrong or unexpected data (like a string instead of a number) and see if your program freaks out or handles it gracefully.
- Check edge cases: Test the boundaries, like the biggest or smallest number you can think of, or what happens if there’s no data at all.
- Ask a friend: A fresh set of eyes will almost always find something you missed.
Here’s a real fact: According to a GitHub report, around 70% of bugs in open-source projects were caught by automated tests, not by people. That’s huge—it means automation isn’t just for pros, it actually saves beginners tons of time too.
Testing Type | Purpose | Tools/Examples |
---|---|---|
Manual Testing | Basic checks by hand | Running code, clicking buttons |
Automated Testing | Repeats checks with scripts | Jest, PyTest, JUnit |
User Testing | Real people try your app | Beta testers, feedback surveys |
The golden rule? Don’t assume your program works just because you want it to. Good testing makes your life and coding classes easier. Think of it as insurance for your project. Even if you’re a beginner, start testing early and often—the payoff comes fast.
If there's one step you don't want to skip in the coding steps process, it's this one.
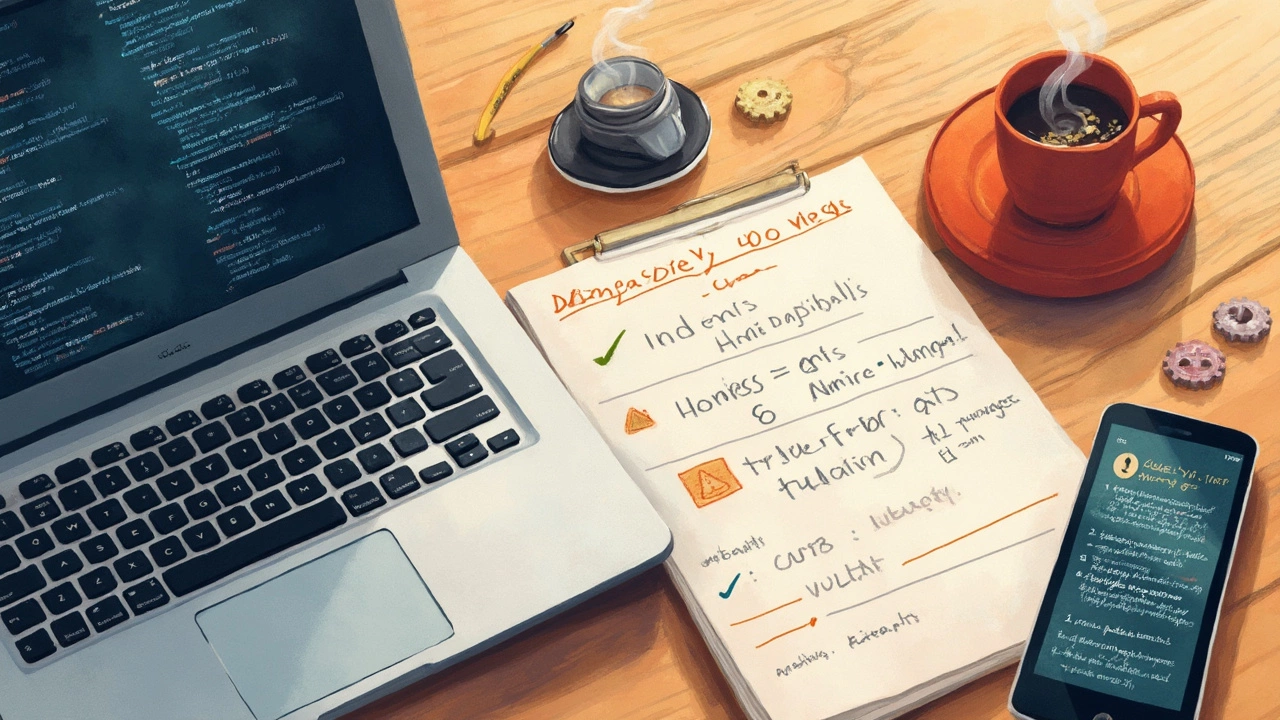
Keeping Your Code Fresh and Updated
You can write the cleanest program ever, but if you ignore it after that, things are going to break down. Software changes, people find better ways to do things, and weird bugs pop up that no one saw coming. This is why keeping your code updated is actually one of the most underrated steps in the coding steps process.
Here’s what works in the real world: first, set up reminders to check your code from time to time. Every few months, glance over your project and see if there are new versions of the tools or frameworks you used. For example, if you're working with React or Python, updates come out regularly. Developers who pay attention to these are usually the ones who avoid running into weird errors down the line.
Bug fixes are another thing you can’t ignore. When someone finds a bug, don’t just patch it quickly and forget about it. Log those issues somewhere, whether it's in a simple spreadsheet or a tool like GitHub Issues. The more you track, the easier it’ll be to spot patterns and clean up your code later.
Another smart habit: write your code so it’s easy to upgrade. This means adding comments, breaking big files into smaller pieces, and using clear names for everything. When you (or someone else) come back in six months, you’ll actually know what’s going on instead of trying to decode your own work like a secret message.
Quick tips to keep code up-to-date:
- Turn on update notifications for frameworks and libraries you use.
- Regularly run automated tests to catch sneaky bugs when things change.
- Clean up old code and delete stuff you don’t need every so often.
- Keep a changelog, even if it’s just a simple text file, to track what you’ve changed.
- Read up on best practices in online communities or official docs—trends in coding change faster than you’d think.
Staying on top isn’t about rewriting your whole project every time something new comes out. It’s more about doing small, regular check-ins and taking action before problems snowball. Trust me—your future self and anyone else touching your code will appreciate the effort.